Memory Retrieval
With Zep's Chat History features you can ensure that your assistant's responses are always relevant to the conversation.
The discussion below does not yet include Zep’s new Graph API. For customers with Graph-enabled accounts, please refer to the Graph Guide for more information.
The Zep Memory API
provides a high-level interface for persisting and retrieving memory artifacts such as Messages, Sessions, and Facts.
The Memory API
is an opinionated, Session-specific API.
Looking for more control over what artifacts are returned or retrieval of artifacts from across multiple Sessions? Explore using Zep’s Search API
Python
TypeScript
Go
Review the Memory Get API documentation for additional arguments and options.
The Memory
API returns Facts relevant to the current conversation and the most recent messages in a conversation.
The number of most recent messages can be configured via the last_n
call argument.
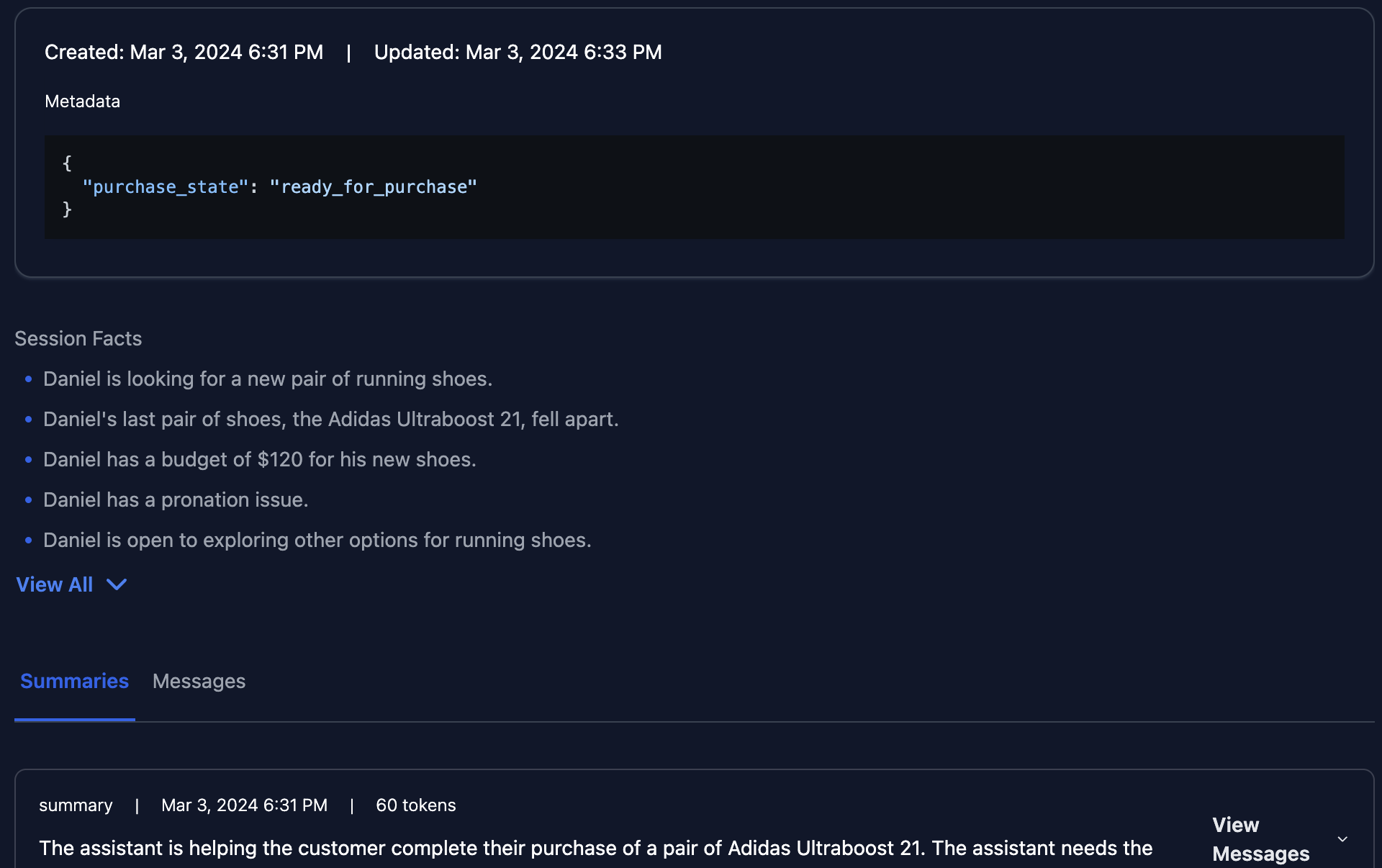